Golf Tracker - Code Templates - Part 3
Making database updates
In the last episode I showed how to modify the views to customize them to my liking, but I didn't actually prove that and database interaction actually works. In this episode I quickly enable the Insert and Update functionality in the repositories and services in order to show you UI interaction.The first thing I need to do is finish writing the code for the repository Insert, Update and GetByID methods. I'll just copy the code from the CourseRepository class into the PlayerRepository class.
These methods will look like this...
01.
public
GolfTracker.BusinessObjects.Player GetById(Guid id)
02.
{
03.
using
(Database db = DataContextFactory.CreateContext())
04.
{
05.
return
PlayerMapper.ToBusinessObject(
06.
db.Players.Where(c => c.ID == id)
07.
.SingleOrDefault());
08.
}
09.
}
10.
11.
public
Guid Insert(GolfTracker.BusinessObjects.Player model)
12.
{
13.
GolfTracker.DataObjects.LinqToSql.Player entity = PlayerMapper.ToEntity(model);
14.
15.
using
(Database db = DataContextFactory.CreateContext())
16.
{
17.
try
18.
{
19.
db.Players.InsertOnSubmit(entity);
20.
db.SubmitChanges();
21.
22.
model.ID = entity.ID;
23.
model.rowversion = VersionConverter.ToString(entity.rowversion);
24.
return
entity.ID;
25.
}
26.
catch
(ChangeConflictException)
27.
{
28.
foreach
(ObjectChangeConflict conflict
in
db.ChangeConflicts)
29.
{
30.
conflict.Resolve(RefreshMode.KeepCurrentValues);
31.
}
32.
try
33.
{
34.
db.SubmitChanges();
35.
}
36.
catch
(ChangeConflictException)
37.
{
38.
throw
new
Exception(
"A concurrency error occurred!"
);
39.
}
40.
return
entity.ID;
41.
}
42.
catch
(Exception)
43.
{
44.
throw
new
Exception(
"There was an error inserting the record!"
);
45.
}
46.
}
47.
}
48.
49.
public
Guid Update(GolfTracker.BusinessObjects.Player model)
50.
{
51.
GolfTracker.DataObjects.LinqToSql.Player entity = PlayerMapper.ToEntity(model);
52.
53.
using
(Database db = DataContextFactory.CreateContext())
54.
{
55.
try
56.
{
57.
db.Players.Attach(entity,
true
);
58.
db.SubmitChanges();
59.
60.
model.ID = entity.ID;
61.
model.rowversion = VersionConverter.ToString(entity.rowversion);
62.
return
entity.ID;
63.
}
64.
catch
(ChangeConflictException)
65.
{
66.
foreach
(ObjectChangeConflict conflict
in
db.ChangeConflicts)
67.
{
68.
conflict.Resolve(RefreshMode.KeepCurrentValues);
69.
}
70.
try
71.
{
72.
db.SubmitChanges();
73.
}
74.
catch
(ChangeConflictException)
75.
{
76.
throw
new
Exception(
"A concurrency error occurred!"
);
77.
}
78.
return
entity.ID;
79.
}
80.
catch
(Exception)
81.
{
82.
throw
new
Exception(
"There was an error updating the record!"
);
83.
}
84.
}
85.
}
Now I need to update the service class.
01.
public
GolfTracker.BusinessObjects.Player GetById(Guid id)
02.
{
03.
return
PlayerRepository.GetById(id);
04.
}
05.
06.
public
Guid Insert(GolfTracker.BusinessObjects.Player model)
07.
{
08.
return
PlayerRepository.Insert(model);
09.
}
10.
11.
public
Guid Update(GolfTracker.BusinessObjects.Player model)
12.
{
13.
return
PlayerRepository.Update(model);
14.
}
Once these are done, then interaction with the PlayerController will work without issues.
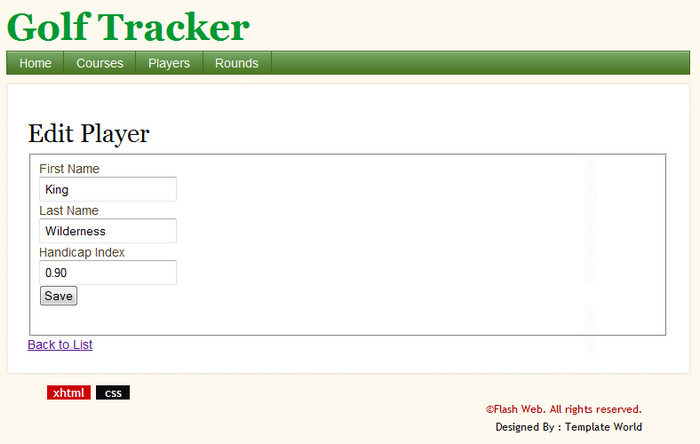
Stay tuned.